Hyperf-Validate
基于hyperf/validation
实现的validate层
安装
composer require death_satan/hyperf-validate -vvv
使用方式
通过命令生成一个 validate.我这里生成一个Demo
php bin/hyperf.php gen:validate Demo
执行后会在你的项目 app/validate目录下生成一个Demo验证器类
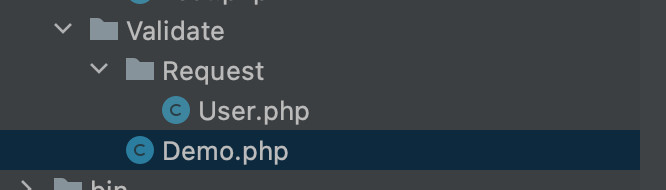
这里我修改为我需要的规则以及场景
<?php
declare(strict_types=1);
namespace App\Validate;
use DeathSatan\Hyperf\Validate\Lib\AbstractValidate as BaseValidate;
class Demo extends BaseValidate
{
protected $scenes =[
'login'=>[
'username','password'
],
'reg'=>[
'username',
],
];
protected function messages():array
{
return [
'username.required'=>'昵称必须填写',
'password.required'=>'密码必须填写'
];
}
protected function attributes():array
{
return [];
}
protected function rules():array
{
return [
'username'=>'required',
'password'=>'required',
];
}
}
在控制器中使用
<?php
declare(strict_types=1);
namespace App\Controller;
use App\Validate\Demo;
use DeathSatan\Hyperf\Validate\Exceptions\ValidateException;
use Hyperf\Di\Annotation\Inject;
use Hyperf\HttpServer\Annotation\AutoController;
use Hyperf\HttpServer\Contract\RequestInterface;
use Hyperf\HttpServer\Contract\ResponseInterface;
class Index
{
protected $validate;
public function index(RequestInterface $request, ResponseInterface $response)
{
$data = $request->all();
try {
$this->validate->scene('login')
->make($data);
}catch (ValidateException $exception)
{
return $response->json([
'msg'=>$exception->getMessage(),//通过getMessage方法获取错误信息
]);
}
return $response->json([
'msg'=>'success'
]);
}
}
测试
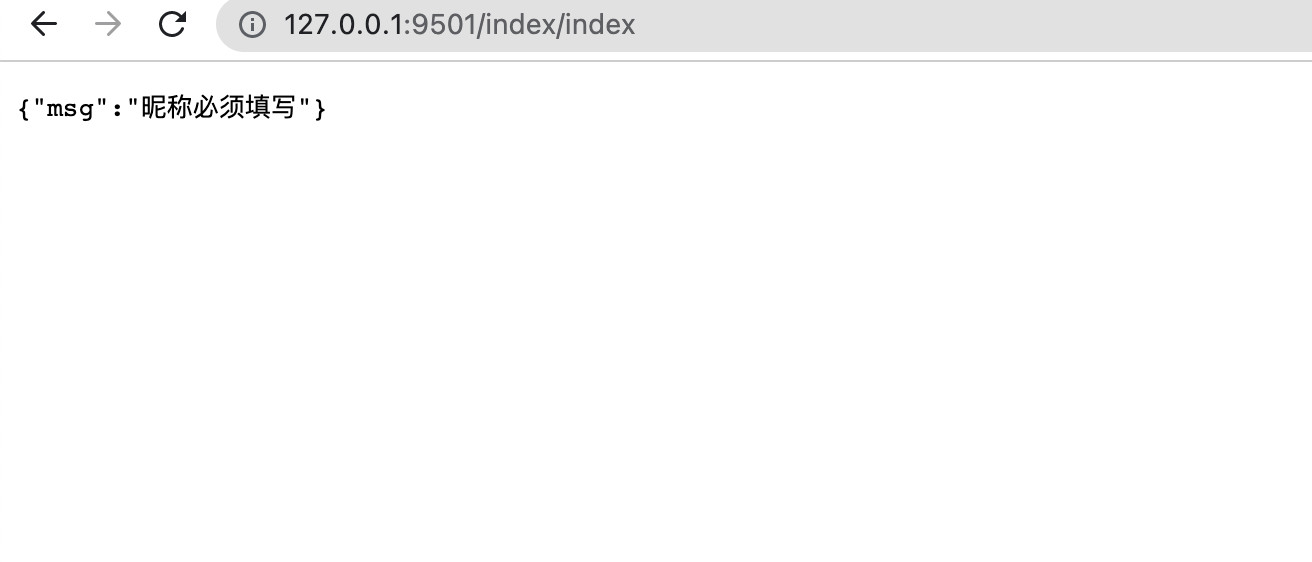
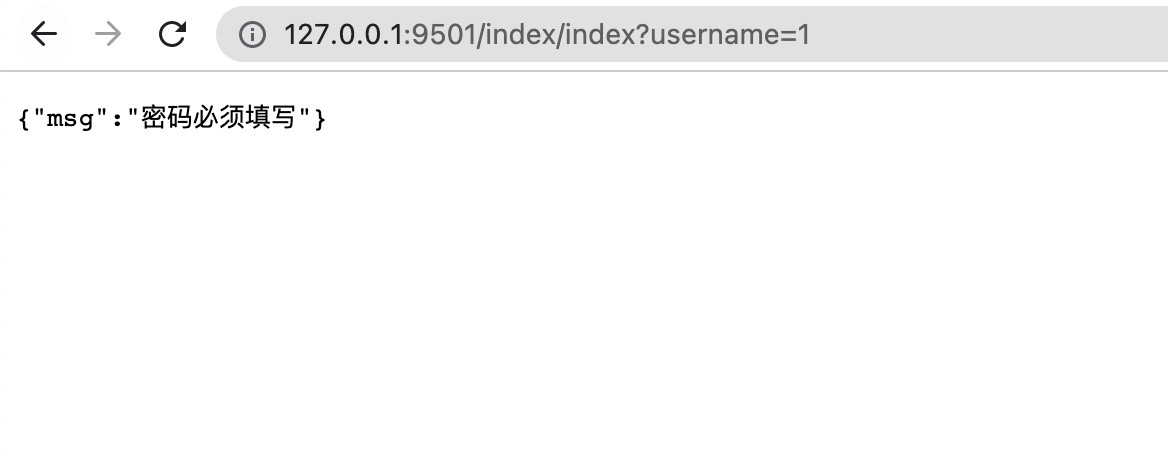
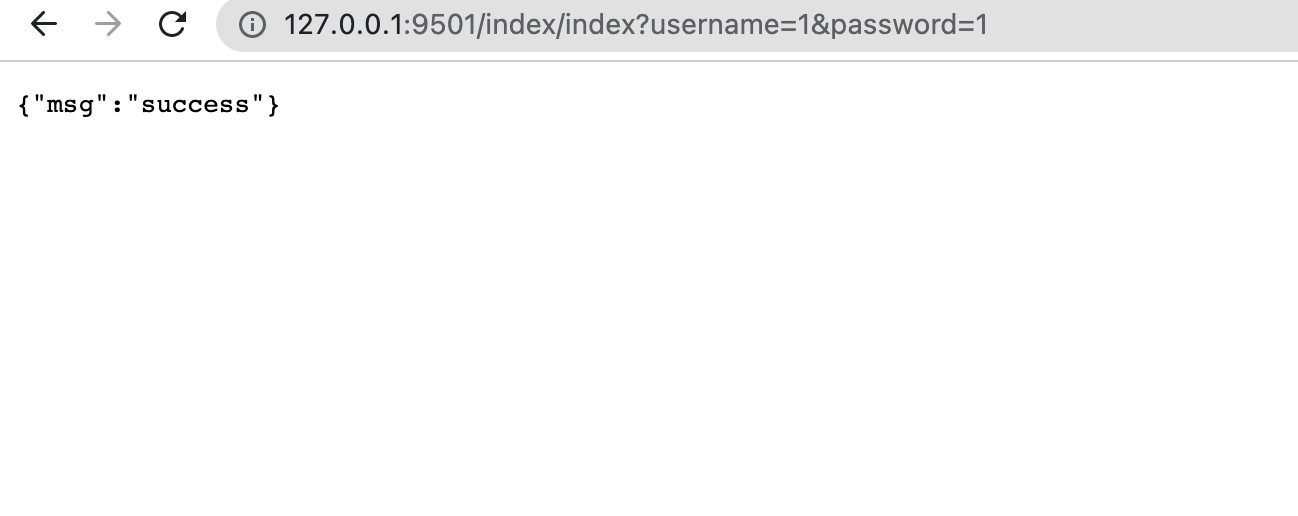
在model中使用
新创建一个表
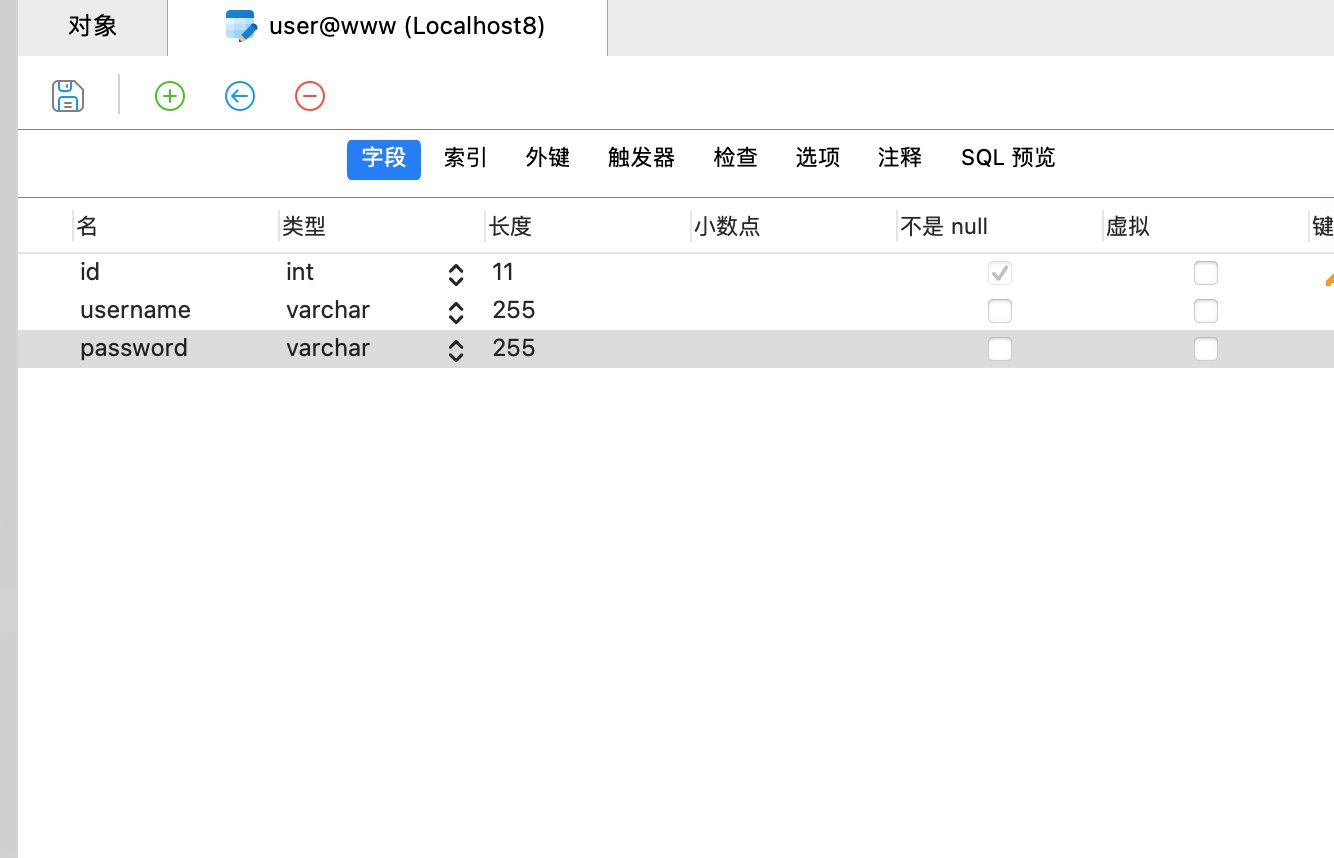
通过gen:model user
生成model, 在model内使用注解标注
<?php
declare (strict_types=1);
namespace App\Model;
use DeathSatan\Hyperf\Validate\Annotation\ModelValidate;
use Hyperf\DbConnection\Model\Model;
class User extends Model
{
protected $table = 'user';
protected $fillable = [
'username','password'
];;
protected $casts = ['id' => 'integer'];
}
在控制器内写入相关代码
<?php
declare(strict_types=1);
namespace App\Controller;
use App\Model\User;
use App\Validate\Demo;
use DeathSatan\Hyperf\Validate\Exceptions\ValidateException;
use Hyperf\Di\Annotation\Inject;
use Hyperf\HttpServer\Annotation\AutoController;
use Hyperf\HttpServer\Contract\RequestInterface;
use Hyperf\HttpServer\Contract\ResponseInterface;
use DeathSatan\Hyperf\Validate\Exceptions\ModelValidateException;
class Index
{
protected $validate;
public function index(RequestInterface $request, ResponseInterface $response)
{
$data = $request->all();
try {
User::create($data);
}catch (ModelValidateException $exception)
{
return $response->json([
'msg'=>$exception->getMessage(),//通过getMessage方法获取错误信息
]);
}
return $response->json([
'msg'=>'success'
]);
}
}
测试
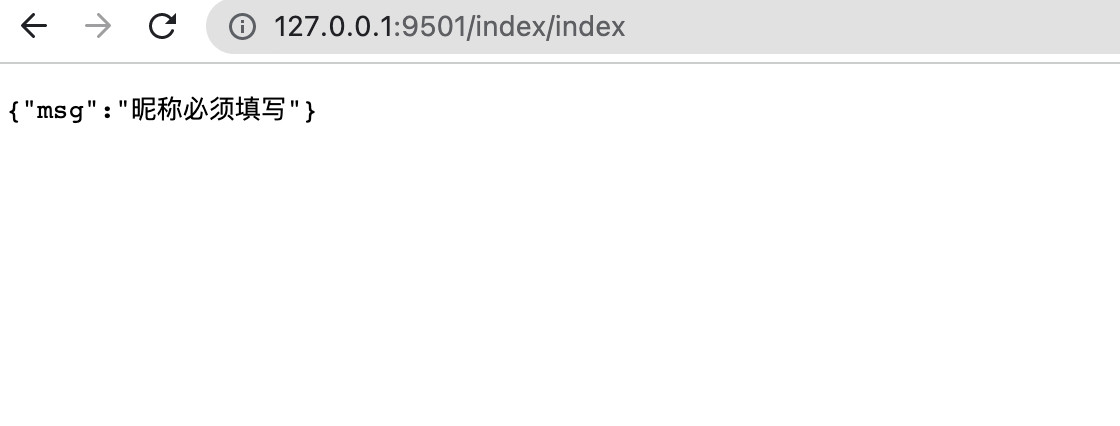
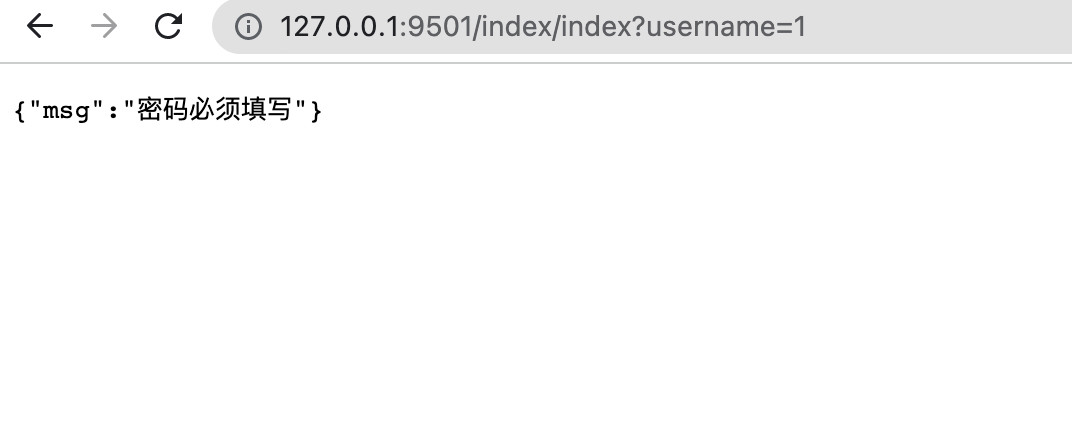
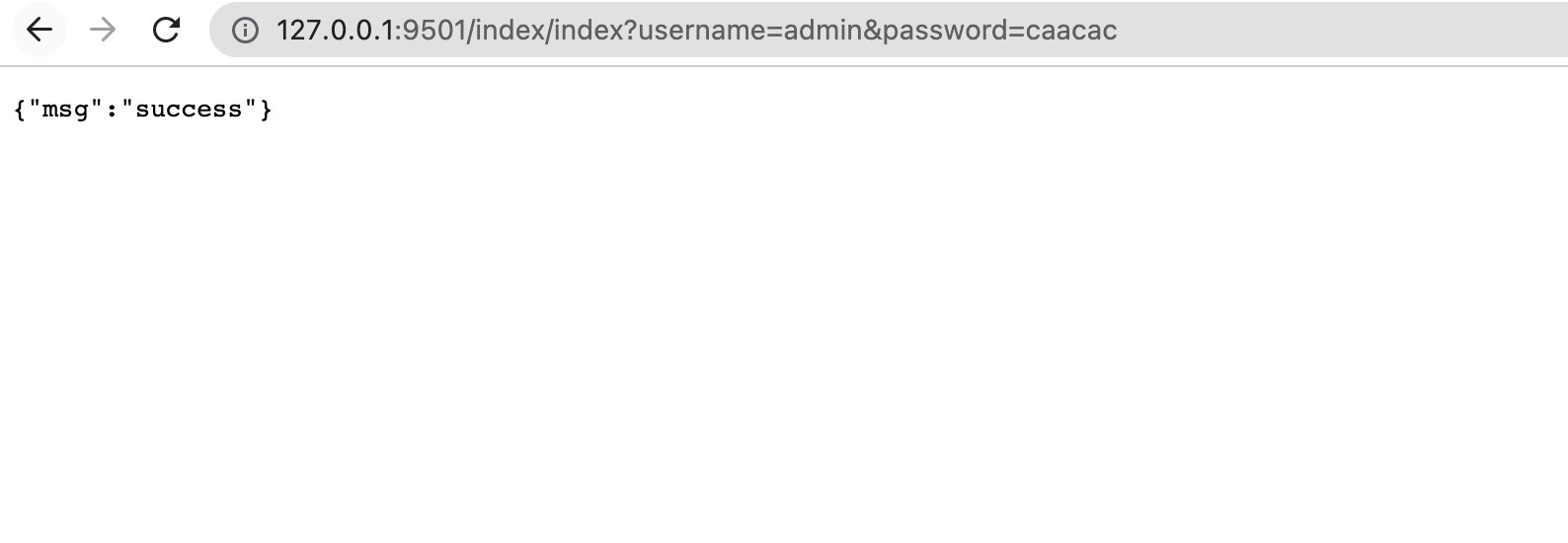
查看数据表
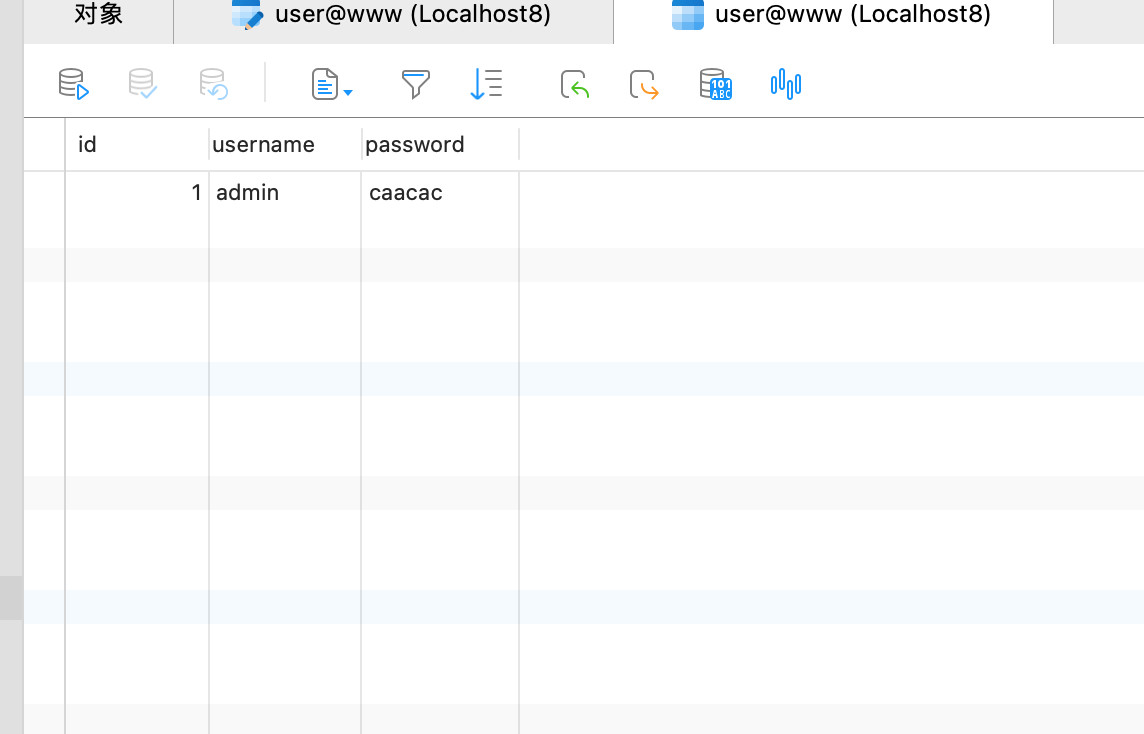
ModelValidate注解参数详解
validate
必选 验证器类名scene
非必选 场景选择event
要在什么事件内进行数据校检 [非必选] 默认:creating,updating,saving